İstediğiniz kaynak kodu yükleniyor!
Yüklemede…
Her şey hazırlandı
Tek yapman gereken yapıştırıp çalıştırmak.
Veri Yapıları Ağaç Yapısı
//Omer Alp KIRAY//
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
struct tree {
int info;
struct tree *left;
struct tree *right;
};
struct tree *Ekleme(struct tree *, int);
void inorder(struct tree *);
void postorder(struct tree *);
void preorder(struct tree *);
struct tree *Silme(struct tree *, int);
struct tree *Arama(struct tree *);
int main(void) {
struct tree *root;
int choice, item, item_no;
root = NULL;
do {
do {
printf("\n1. Eleman Ekle ");
printf("\n2. Eleman Silme ");
printf("\n3. Preorder Siralama ");
printf("\n4. Inorder Siralama ");
printf("\n5. Postorder Siralama ");
printf("\n6. Eleman Degistir ");
printf("\n7. Cikis ");
printf("\nSecim: ");
scanf_s("%d", &choice);
if (choice < 1 || choice>7) {
printf("\n Yanlis Secim");
}
} while (choice < 1 || choice>7);
switch(choice) {
case 1:
printf("\nYeni eleman ekle:");
scanf_s("%d", &item);
root = Ekleme(root, item);
/*printf(\n Kok: %d",root->info);
printf("\n Inorder Siralama: ");
inorder(root);*/
break;
case 2:
printf("\nSilinecek Eleman: ");
scanf_s("%d", &item_no);
root = Silme(root, item_no);
inorder(root);
break;
case 3:
printf("\nPreorder Siralama: ");
preorder(root);
break;
case 4:
printf("\nInorder Siralama: ");
inorder(root);
break;
case 5:
printf("\nPostorder Siralama: ");
postorder(root);
break;
case 6:
printf("\nBul ve Yer Degistir");
root = Arama(root);
break;
default:
printf("\nProgram Cikisi");
}/*Switch Sonu*/
} while (choice != 7);
return(0);
}
/* Eleman Ekleme Fonksiyonu*/
struct tree *Ekleme(struct tree *root,int x)
{
if (!root) {
root = (struct tree*)malloc(sizeof(struct tree));
root->info = x;
root->left = NULL;
root->right = NULL;
return(root);
}
if (root->info > x) {
root->left = Ekleme(root->left, x);
}
else {
if (root->info < x) {
root->right = Ekleme(root->right, x);
}
}
return(root);
}
/* Inorder Fonksiyonu */
void inorder(struct tree *root) {
if (root != NULL) {
inorder(root->left);
printf("%d\t", root->info);
inorder(root->right);
}
return;
}
/* Postorder Fonksiyonu */
void postorder(struct tree *root) {
if (root != NULL) {
postorder(root->left);
postorder(root->right);
printf("%d\t", root->info);
}
return;
}
/* Preorder Fonksiyonu */
void preorder(struct tree *root) {
if (root != NULL) {
printf("%d\t", root->info);
preorder(root->left);
preorder(root->right);
}
return;
}
/* Silme Fonksiyonu*/
struct tree *Silme(struct tree *ptr, int x) {
struct tree *p1, *p2;
if (!ptr) {
printf("\nEleman Bulunamadi\n");
return(ptr);
}
else {
if (ptr->info < x) {
ptr->right = Silme(ptr->right, x);
}
else if (ptr->info > x) {
ptr->left = Silme(ptr->left, x);
return ptr;
}
else /*no. 2 else*/
{
if (ptr->info == x) { /* no 2 if */
if (ptr->left == ptr->right) { /* i.e., a leaf node */
free(ptr);
return NULL;
}
else if (ptr->left == NULL) { /* a right subtree */
p1 = ptr->right;
free(ptr);
return p1;
}
else if (ptr->right == NULL) { /* a left subtree*/
p1 = ptr->left;
free(ptr);
return p1;
}
else {
p1 = ptr->right;
p2 = ptr->right;
while (p1->left != NULL) {
p1 = p1->left;
}
p1->left = ptr->left;
free(ptr);
return p2;
}
} /* end of no. 2 if*/
} /* end of no. 2 else*/
}
return(ptr);
}
/* Arama ve Yer Degistirme Fonksiyonu*/
struct tree *Arama(struct tree *root) {
int no, i, ino;
struct tree *ptr;
ptr = root;
printf("\n Aranan Eleman : ");
scanf_s("%d", &no);
fflush(stdin);
while (ptr) {
if (no > ptr->info) {
ptr = ptr->right;
}
else if (no < ptr->info) {
ptr = ptr->left;
}
else {
break;
}
}
if (ptr) {
printf("\nEleman %d Agacta bulundu = %d", no, ptr->info);
printf("\nDegistirmek icin 1'e Basin");
scanf_s("%d", &i);
if (i == 1) {
printf("\nYeni Eleman Ekle:");
scanf_s("%d", &ino);
ptr->info = ino;
}
else {
printf("\nIslem Iptal...");
}
}
else {
printf("\nEleman %d Agacta Bulunamadi", no);
}
return root;
}
Bilgisayar mühendisliği dersinde verilen Veri Yapıları dersi içerisinde oluşturulmuş: Ağaç veri yapısıdır. Ekran Çıktısı:
Menü: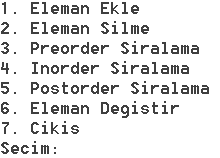